XSLT is very efficient to transform XML documents into another XML documents, and since C# coding and using .NET assemblies, it became more powerful, leading to make XSLT widely used within the integrations so far.
Integrations are implemented to manage dataflow between the source systems and the destination systems, some systems still generate XML as their main output format for the data being transferred, while others use JSON format as their input/output, in such cases, integrations are required besides mapping the data between the two systems, to also reformat them in the accepted format for the destination systems.
XSLT can be used to generate JSON output from an XML input, to do this, the method attribute for the output should be changed to text.
<xsl:output method="text" indent="yes" />
Let’s consider the following example:
You want to synchronize your website’s database for the available movies, the source system sends the movies data in XML format as the following:
<Movies>
<Movie name="The Shawshank Redemption" release="1994" director="Frank Darabont">
<Actors>
<Actor>Morgan Freeman</Actor>
<Actor>Tim Robbins</Actor>
<Actor>William Sadler</Actor>
</Actors>
</Movie>
<Movie name="L.A. Confidential" release="1997" director="Curtis Hanson">
<Actors>
<Actor>Kevin Spacey</Actor>
<Actor>Russell Crowe</Actor>
<Actor>Kim Basinger</Actor>
</Actors>
</Movie>
<Movie name="Basic Instinct" release="1992" director="Paul Verhoeven">
<Actors>
<Actor>Michael Douglas</Actor>
<Actor>Sharon Stone</Actor>
<Actor>Jeanne Tripplehorn</Actor>
</Actors>
</Movie>
</Movies>
Your website (the destination system) expects to receive the data in JSON format as the following:
[
{
"name": "The Shawshank Redemption",
"year": "1994",
"director": "Frank Darabont",
"actors": [
"Morgan Freeman",
"Tim Robbins",
"William Sadler"
]
},
{
"name": "L.A. Confidential",
"year": "1997",
"director": "Curtis Hanson",
"actors": [
"Kevin Spacey",
"Russell Crowe",
"Kim Basinger"
]
},
{
"name": "Basic Instinct",
"year": "1992",
"director": "Paul Verhoeven",
"actors": [
"Michael Douglas",
"Sharon Stone",
"Jeanne Tripplehorn"
]
}
]
You can use XSLT to do this transformation and to map the source fields to the destinations
<?xml version="1.0" encoding="utf-8"?>
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform"
xmlns:msxsl="urn:schemas-microsoft-com:xslt" exclude-result-prefixes="msxsl"
>
<xsl:output method="text" indent="yes"/>
<xsl:template match="/">
<xsl:apply-templates select="/Movies" />
</xsl:template>
<xsl:template match="Movies">
<xsl:text>[</xsl:text>
<xsl:for-each select="Movie">
<xsl:if test="position()>1">
<xsl:text>,</xsl:text>
</xsl:if>
<xsl:text>{</xsl:text>
<xsl:text>"name": "</xsl:text>
<xsl:value-of select="@name"/>
<xsl:text>", "year": "</xsl:text>
<xsl:value-of select="@release"/>
<xsl:text>", "director": "</xsl:text>
<xsl:value-of select="@director"/>
<xsl:text>", "actors":[</xsl:text>
<xsl:for-each select="Actors/Actor">
<xsl:if test="position()>1">
<xsl:text>,</xsl:text>
</xsl:if>
<xsl:text>"</xsl:text>
<xsl:value-of select="."/>
<xsl:text>"</xsl:text>
</xsl:for-each>
<xsl:text>]</xsl:text>
<xsl:text>}</xsl:text>
</xsl:for-each>
<xsl:text>]</xsl:text>
</xsl:template>
</xsl:stylesheet>
This map can be used in a logic app (standard or consumption), and if it is necessary, it can be converted to a json object so easy
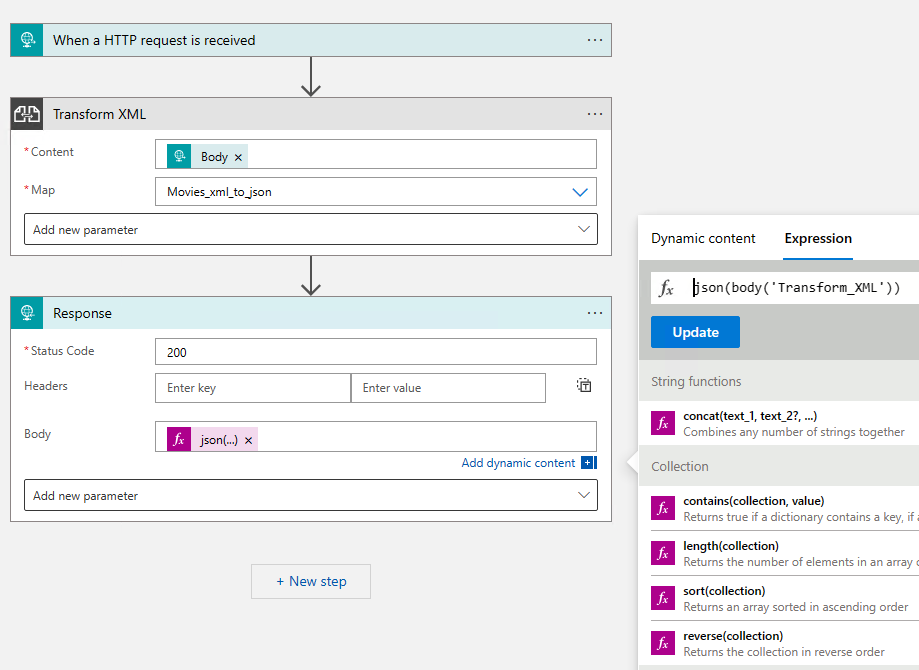
Why to use XSLT?
If the input is a complex XML or requires data processing, XSLT is a convenient transformation language compared to Liquid. It allows writing inline C# functions or even calling custom assemblies if necessary.
Leave a Reply